API Reference
The following content is for DocSearch v3. If you are using DocSearch v2, see the legacy documentation.
- JavaScript
- React
container
type: string | HTMLElement
| required
The container for the DocSearch search box. You can either pass a CSS selector or an Element. If there are several containers matching the selector, DocSearch picks up the first one.
environment
type: typeof window
|default: window
| optional
The environment in which your application is running.
This is useful if you’re using DocSearch in a different context than window.
appId
type: string
| required
Your Algolia application ID.
apiKey
type: string
| required
Your Algolia Search API key.
indexName
type: string
| required
Your Algolia index name.
placeholder
type: string
|default: "Search docs"
| optional
The placeholder of the input of the DocSearch pop-up modal.
searchParameters
type: SearchParameters
| optional
The Algolia Search Parameters.
transformItems
type: function
|default: items => items
| optional
Receives the items from the search response, and is called before displaying them. Should return a new array with the same shape as the original array. Useful for mapping over the items to transform, and remove or reorder them.
- JavaScript
- React
docsearch({
// ...
transformItems(items) {
return items.map((item) => ({
...item,
content: item.content.toUpperCase(),
}));
},
});
<DocSearch
// ...
transformItems={(items) => {
return items.map((item) => ({
...item,
content: item.content.toUpperCase(),
}));
}}
/>
hitComponent
type: ({ hit, children }) => JSX.Element
|default: Hit
| optional
The component to display each item.
See the default implementation.
transformSearchClient
type: function
|default: searchClient => searchClient
| optional
Useful for transforming the Algolia Search Client, for example to debounce search queries
disableUserPersonalization
type: boolean
|default: false
| optional
Disable saving recent searches and favorites to the local storage.
initialQuery
type: string
| optional
The search input initial query.
navigator
type: Navigator
| optional
An implementation of Algolia Autocomplete’s Navigator API to redirect the user when opening a link.
Learn more on the Navigator API documentation.
translations
type: Partial<DocSearchTranslations>
|default: docSearchTranslations
| optional
Allow translations of any raw text and aria-labels present in the DocSearch button or modal components.
docSearchTranslations
const translations: DocSearchTranslations = {
button: {
buttonText: 'Search',
buttonAriaLabel: 'Search',
},
modal: {
searchBox: {
resetButtonTitle: 'Clear the query',
resetButtonAriaLabel: 'Clear the query',
cancelButtonText: 'Cancel',
cancelButtonAriaLabel: 'Cancel',
searchInputLabel: 'Search',
},
startScreen: {
recentSearchesTitle: 'Recent',
noRecentSearchesText: 'No recent searches',
saveRecentSearchButtonTitle: 'Save this search',
removeRecentSearchButtonTitle: 'Remove this search from history',
favoriteSearchesTitle: 'Favorite',
removeFavoriteSearchButtonTitle: 'Remove this search from favorites',
},
errorScreen: {
titleText: 'Unable to fetch results',
helpText: 'You might want to check your network connection.',
},
footer: {
selectText: 'to select',
selectKeyAriaLabel: 'Enter key',
navigateText: 'to navigate',
navigateUpKeyAriaLabel: 'Arrow up',
navigateDownKeyAriaLabel: 'Arrow down',
closeText: 'to close',
closeKeyAriaLabel: 'Escape key',
searchByText: 'Search by',
},
noResultsScreen: {
noResultsText: 'No results for',
suggestedQueryText: 'Try searching for',
reportMissingResultsText: 'Believe this query should return results?',
reportMissingResultsLinkText: 'Let us know.',
},
},
};
getMissingResultsUrl
type: ({ query: string }) => string
| optional
Function to return the URL of your documentation repository.
- JavaScript
- React
docsearch({
// ...
getMissingResultsUrl({ query }) {
return `https://github.com/algolia/docsearch/issues/new?title=${query}`;
},
});
<DocSearch
// ...
getMissingResultsUrl={({ query }) => {
return `https://github.com/algolia/docsearch/issues/new?title=${query}`;
}}
/>
When provided, an informative message wrapped with your link will be displayed on no results searches. The default text can be changed using the translations property.
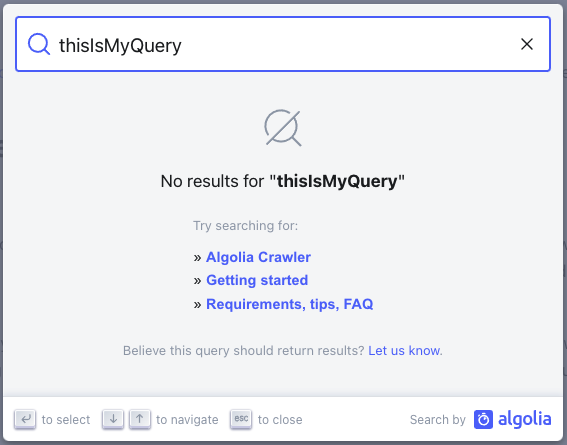
resultsFooterComponent
type: ({ state }) => JSX.Element
| optional
The component to display below the search results.
You get access to the current state which allows you to retrieve the number of hits returned, the query etc.
You can find a working example without JSX in this sandbox.
- JavaScript
- React
docsearch({
// ...
resultsFooterComponent({ state }) {
return {
// The HTML `tag`
type: 'a',
ref: undefined,
constructor: undefined,
key: state.query,
// Its props
props: {
href: 'https://docsearch.algolia.com/apply',
target: '_blank',
onClick: (event) => {
console.log(event);
},
// Raw text rendered in the HTML element
children: `${state.context.nbHits} hits found!`,
},
__v: null,
};
},
});
<DocSearch
// ...
resultsFooterComponent={({ state }) => {
return <h1>{state.context.nbHits} hits found</h1>;
}}
/>
maxResultsPerGroup
type: number
| optional
The maximum number of results to display per search group. Default is 5.
You can find a working example without JSX in this sandbox
- JavaScript
docsearch({
// ...
maxResultsPerGroup: 7,
});